Reading and Writing XML Documents (C++)
After you generate code from the example schema, a test C++ application is created, along with several supporting Altova libraries.
About the generated C++ libraries
The central class of the generated code is the CDoc class, which represents the XML document. Such a class is generated for every schema and its name depends on the schema file name. As shown in the diagram, this class provides methods for loading documents from files, binary streams, or strings (or saving documents to files, streams, strings). For a description of all members exposed by this class, see the class reference ([YourSchema]::[CDoc]).
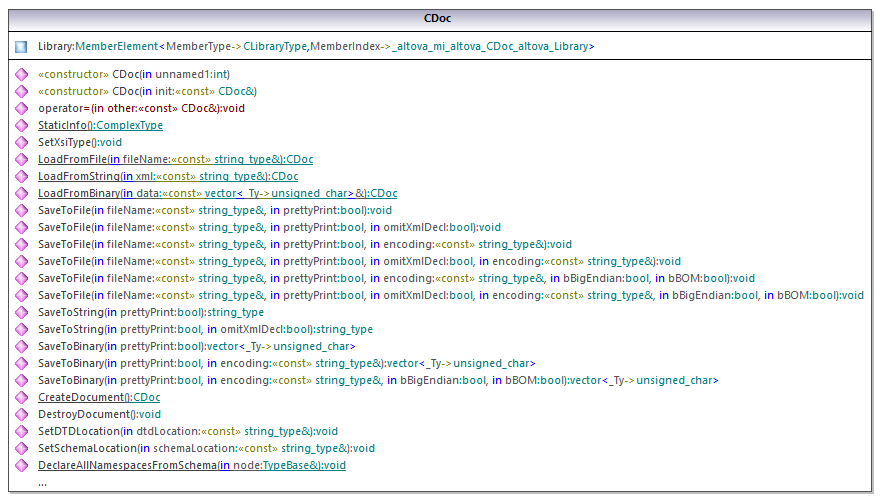
The Library field of the CDoc class represents the actual root of the document. Library is an element in the XML file, so in the C++ code it has a template class as type (MemberElement). The template class exposes methods and properties for interacting with the Library element. In general, each attribute and each element of a type in the schema is typed in the generated code with the MemberAttribute and MemberElement template classes, respectively. For more information, see [YourSchema]::MemberAttribute and [YourSchema]::MemberElement class reference.
The class CLibraryType is generated from the LibraryType complex type in the schema. Notice that the CLibraryType class contains two fields: Book and LastUpdated. According to the logic already mentioned above, these correspond to the Book element and LastUpdated attribute in the schema, and enable you to manipulate programmatically (append, remove, etc) elements and attributes in the instance XML document.
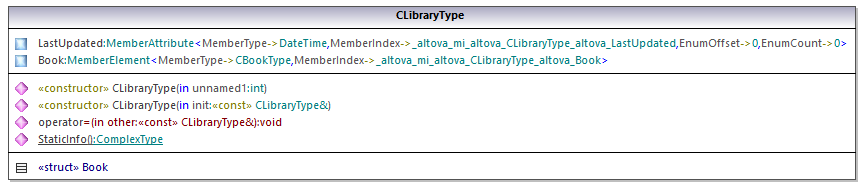
The DictionaryType is a complex type derived from BookType in the schema, so this relationship is also reflected in the generated classes. As illustrated in the diagram, the class CDictionaryType inherits the CBookType class.
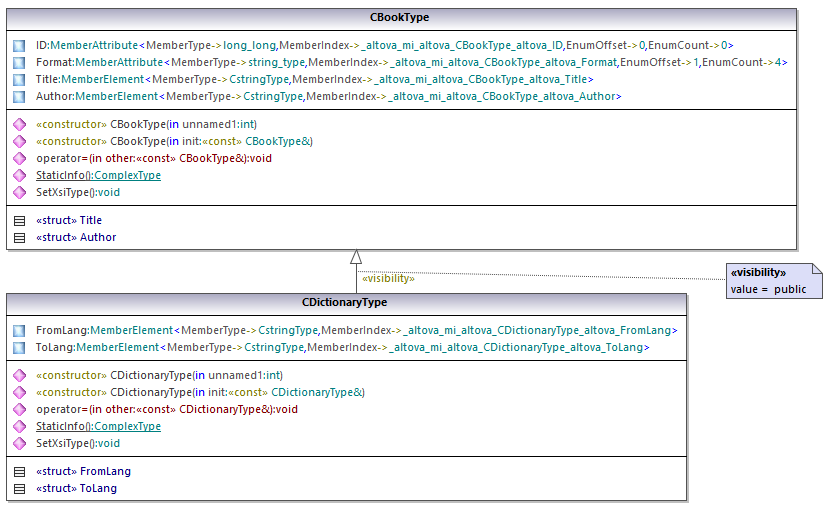
If your XML schema defines simple types as enumerations, the enumerated values become available as enum values in the generated code. In the schema used in this example, a book format can be hardcover, paperback, e-book, and so on. Therefore, in the generated code, these values would be available through an enum that is a member of the CBookFormatType class.
Writing an XML document
1.Open the LibraryTest.sln solution in Visual Studio generated from the Library schema mentioned earlier in this example.
While prototyping an application from a frequently changing XML schema, you may need to frequently generate code to the same directory, so that the schema changes are immediately reflected in the code. Note that the generated test application and the Altova libraries are overwritten every time when you generate code into the same target directory. Therefore, do not add code to the generated test application. Instead, integrate the Altova libraries into your project (see Integrating Schema Wrapper Libraries). |
2.In Solution Explorer, open the LibraryTest.cpp file, and edit the Example() method as shown below.
#include <ctime> // required to get current time |
3.Press F5 to start debugging. If the code was executed successfully, a GeneratedLibrary.xml file is created in the solution output directory.
Reading an XML document
1.Open the LibraryTest.sln solution in Visual Studio.
2.Save the code below as Library1.xml to a directory that can be read by the program code (for example, the same directory as LibraryTest.sln).
<?xml version="1.0" encoding="utf-8"?> |
3.In Solution Explorer, open the LibraryTest.cpp file, and edit the Example() method as shown below.
using namespace Doc; |
4.Press F5 to start debugging.