Retrieving Command Information
The MapForceControl gives access to all commands of MapForce through its CommandsList, MainMenu, and Toolbars properties. The example project available in the folder <ApplicationFolder>\Examples\ActiveX\C# uses the MainMenu property to create the MapForce menu structure dynamically.
The code that gets the menu commands can be found in the MDIMain method in MDIMain.cs file:
public MDIMain() |
In the code listing above, mainMenu is the existing static menu of the main MDI Frame window. If you open the MDIMain.cs form in the Visual Studio Designer, you will notice that this menu contains two menu items: File and Window.
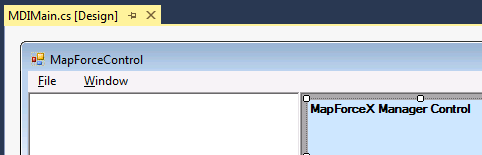
MDIMain.cs
The method InsertMenuStructure takes as parameters the mainMenu and the objCommand objects (the former is the existing static menu, while the latter contains the full menu structure retrieved from the MapForce ActiveX control). The retrieved MapForce menu structure is then merged into the existing static menu. Note that the menus File, Project, and Window are not added dynamically. This is intentional, because these menus deal with actively open documents, and they would require code which is beyond the scope of this example. The basic file management commands (create, open, save, bring into focus) are handled by the existing static menus File and Window. All other menus are inserted dynamically based on the information taken from the MainMenu property of the ActiveX control. The new menus are inserted after "File" but before "Window", i.e. starting at menu index 1.
The method InsertMenuStructure iterates through all top-level menus found in MapForceCommand object and adds a new menu item for each. Since each top-level menu has its own child menu items, a call to the method InsertMenuCommand takes place for each encountered child menu item. Furthermore, since each child menu item can have its own children menu items, and so on, the InsertMenuCommand method recurses into itself until no more child menu items exist.
The commands added dynamically are instances of the class CustomMenuItem, which is defined in CustomMenuItem.cs. This class is derived from System.Windows.Forms.MenuItem class and has an additional member to store the MapForce command ID.
public class CustomMenuItem : System.Windows.Forms.MenuItem { public int m_MapForceCmdID; } |
All dynamically added commands (except those that are containers for other commands) get the same event handler AltovaMenuItem_Click which does the processing of the command:
private void AltovaMenuItem_Click(object sender, EventArgs e) |
If the command is a container for other commands (that is, if it has child commands), it gets the event handler AltovaSubMenu_Popup. This handler queries the status of each child command and enables or disables it as required. This ensures that each command is enabled only when that is meaningful (for example, the File | Save menu item should be disabled if there is no active document open).
The method ProcessCommand delegates the execution either to the MapForceControl itself or to any active MapForce document loaded in a MapForceControlDocument control. This is necessary because the MapForceControl has no way to know which document is currently active in the hosting application.
private void ProcessCommand(int nID) |