How to create Sequence diagrams manually
Creating new Sequence Diagrams programmatically from scratch using the UModel API is basically nothing more than placing interaction fragments, such as Lifelines on a diagram and connecting them with messages.
Messages can easily be created using the AddUMLLineElement() method of IUMLGuiLineLink, which removes the necessity of creating multiple underlying UML Elements such as MessageEnds, ExecutionOccurrences and similar manually.
To make it simple to create Messages between two interaction fragments such as Lifelines, create a small helper function which calls AddUMLLineElement() and positions the created line:
// Creates a message between two interaction fragments (i.e. lifelines, interaction uses, |
As you can see, IUMLDiagram.AddUMLLineElement() accepts as a parameter not only the string "Message", to create a Message Line; but also "Reply", "Create" and "Destruct", for Reply Messages, Creation Messages and Destruction Messages.
In order to create a simple diagram it is only necessary to create a Sequence Diagram in the GuiRoot object, open the diagram, add a handful of lifelines and connect them with messages using this helper function:
IDocument document = theapplication.ActiveDocument; |
The resulting created Diagram will look like this:
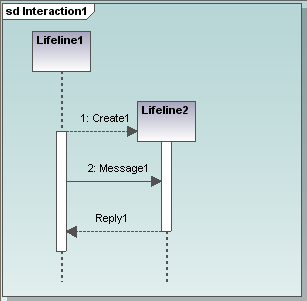
Setting the Type of a Lifeline
To display the Type represented by a Lifeline, be it be a Class, Interface, DataType or similar, use the IUMLLifeline.Represents property which references a IUMLProperty. If the Type of this property is set, the Type will show up on the diagram as well.
The following code creates a Lifeline which references a class:
// create a class to be referenced by the lifeline |
The resulting lifeline would then look like this:
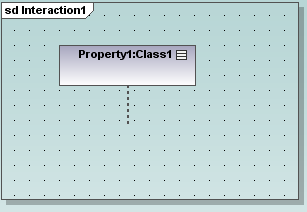
Setting the Operation of a Message
Messages usually represent the invocation of an operation of an object. Note: based on the type of the Message (normal Message, Creation, Deletion or Reply) and the existence, or absence of underlying UML elements, such as MessageOccurenceSpecifications or CallEvents, it is not always possible for a Message to represent an Operation, and getting the cprrect UML element to point to the Operation is not that trivial.
This is why the IUMLMessage interface in the UModel API, offers the method SetOperation() with makes it possible to let a Message refer an Operation if it is able to do so:
// create a message, an operation in a class and let the message refer this operation |