Example: Run Mapping with Parameters
This example shows you how to compile a MapForce mapping to a MapForce Server execution file (.mfx) and run it from the MapForce API. The example specifically illustrates the scenario when the mapping takes the input file name as parameter. See also any of the previous C#, C++, VB.NET, VBScript, or Java examples.
In this example, MapForce is used so that you can view and understand the original mapping design. MapForce is also used to compile the mapping to a MapForce Server execution file (.mfx) and configure settings such as relative versus absolute paths.
The server platform used in the example is Windows. This could be either the same machine where MapForce is installed, or a different one. You can also run this example on a Linux or Mac machine (in Java), provided that you adjust the Windows-style paths as applicable to your platform.
Prerequisites
Running this mapping has the same prerequisites as described in the previous C#, C++, VB.NET, VBScript, or Java examples.
Preparing the mapping for server execution
After installing MapForce and running MapForce for the first time, several demo mapping design files are available at the following path:
C:\Users\<username>\Documents\Altova\MapForce2025\MapForceExamples
Make sure to change the path above accordingly if you have a different version of MapForce.
The mapping design used in this example is called FileNamesAsParameters.mfd. As illustrated below, this is a straightforward mapping that copies data from a source to a target XML file almost unchanged. Only the PrimaryKey and Name fields of the target XML file are populated with constants from the mapping.
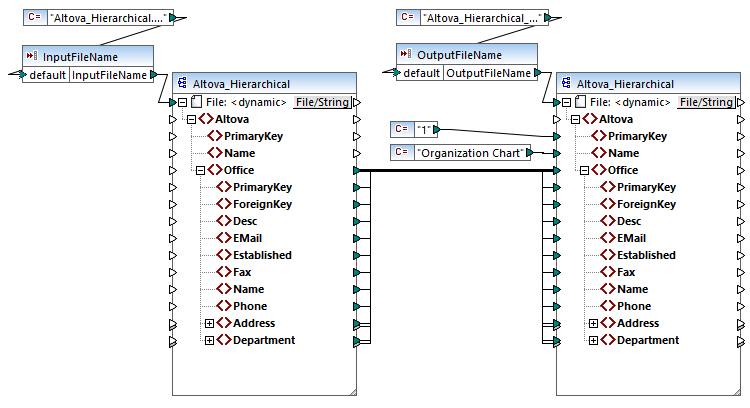
FileNamesAsParameters.mfd
The mapping has two input parameters: InputFileName and OutputFileName, respectively. In order to make it possible to preview the mapping in MapForce, the parameter values are supplied by two constants. As further described below, you will be able to replace the parameter values with your own when the mapping runs with MapForce Server.
Notice that both the source and target mapping components are configured to get the file name dynamically from the mapping. Namely, a File: <dynamic> input connector is available at the very top of the component, and it reads data from the respective mapping parameter (InputFileName or OutputFileName). In MapForce, you can set or change this option by clicking the File/String button in the top-right corner of the component. Be aware that the input file name supplied as parameter must be a reference to a physical XML file that provides input data to the mapping (in this example, Altova_Hierarchical.xml from the same folder as the mapping design file). The output file name can be any valid name, for example Output.xml.
Before compiling the mapping to a MapForce Server Execution file (.mfx), you will typically want to review the mapping settings. Right-click on an empty area on the mapping, and select Mapping Settings from the context menu.
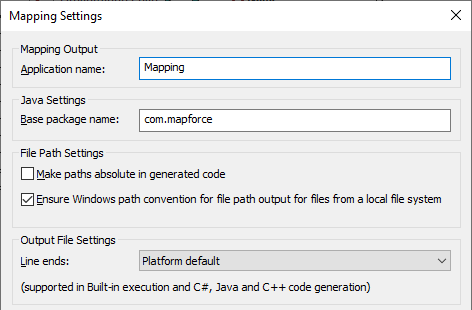
For the scope of this example, change the settings as illustrated above. Specifically, when you clear the Make paths absolute in generated code check box, any absolute paths to input or output files used by the mapping are resolved as relative to the mapping design file (.mfd). At mapping runtime, MapForce Server will look for these paths in the program's working directory. The default working directory depends on the platform that you use to access the MapForce Server API. For example, in case of a C# application, it is the same directory as the executable. In addition, as further illustrated below, you can change the working directory with the help of an API property called WorkingDirectory (or setWorkingDirectory Java method, if applicable).
Notes:
•On the Mapping Settings dialog box, the only settings that directly affect the compilation of the .mfx file are Make paths absolute in generated code and Line ends.
•In this mapping, we did not need to edit each mapping component in MapForce so as to change absolute paths to relative because all paths were already relative. Otherwise, you would need to perform this extra step as well, see Preparing Mappings for Server Execution.
In this example, we will use C:\MappingExample as working directory. Therefore, copy the input file Altova_Hierarchical.xml referenced by the mapping to from C:\Users\<username>\Documents\Altova\MapForce2025\MapForceExamples to the working directory.
In this example, both the source and target are XML files, and there is no need to copy the XML schema file to the server, because information derived from it is embedded into the .mfx file during compilation. If your mapping uses other component types (for example, databases), there could be additional prerequisites, as described in Preparing Mappings for Server Execution.
Finally, to compile the mapping to a server execution file, do the following:
•On the File menu, click Compile to MapForce Server Execution file and select a target directory. In this example, the target directory is the same as working directory, C:\MappingExample.
The following code listings illustrate how to run the mapping from various environments, using the MapForce Server API. In the code listings below, notice that both parameters are referenced by the same name that they have in the mapping design. Also, the parameter value has the same data type as defined on the mapping (in this case, string).
Running the mapping from C++
You can now run the .mfx file by using C++ code such as the one below. To run this code listing successfully, make sure that MapForce Server has a valid license, see also the verifylicense CLI command.
C++
#include <iostream> |
Running the mapping from C#
You can now run the .mfx file by using C# code such as the one below. To run this code listing successfully, first add a reference to the MapForce Server DLL in Visual Studio, as described in .NET Interface, and make sure that MapForce Server has a valid license, see also the verifylicense CLI command.
C#
static void Main(string[] args) |
Running the mapping from Java
You can now run the .mfx file by using Java code such as the one below. To run this code listing successfully, make sure that:
•the Java CLASSPATH environment variable includes a reference to the MapForceServer.jar library, as described in About the Java Interface
•MapForce Server has a valid license, see also the verifylicense CLI command.
Java
public static void main(String[] args) { |